Transformer¶
Create Function¶
Transformers can be either created from the standard type library (create_transformer) or with custom values (create_transformer_from_parameters).
-
pandapower.
create_transformer
(net, hv_bus, lv_bus, std_type, name=None, tp_pos=nan, in_service=True, index=None, max_loading_percent=nan, parallel=1, df=1.)¶ Creates a two-winding transformer in table net[“trafo”]. The trafo parameters are defined through the standard type library.
- INPUT:
net - The net within this transformer should be created
hv_bus (int) - The bus on the high-voltage side on which the transformer will be connected to
lv_bus (int) - The bus on the low-voltage side on which the transformer will be connected to
std_type - The used standard type from the standard type library
- OPTIONAL:
name (string, None) - A custom name for this transformer
tp_pos (int, nan) - current tap position of the transformer. Defaults to the medium position (tp_mid)
in_service (boolean, True) - True for in_service or False for out of service
index (int, None) - Force a specified ID if it is available. If None, the index one higher than the highest already existing index is selected.
max_loading_percent (float) - maximum current loading (only needed for OPF)
parallel (integer) - number of parallel transformers
df (float) - derating factor: maximal current of transformer in relation to nominal current of transformer (from 0 to 1)
- OUTPUT:
- index (int) - The unique ID of the created transformer
- EXAMPLE:
- create_transformer(net, hv_bus = 0, lv_bus = 1, name = “trafo1”, std_type = “0.4 MVA 10/0.4 kV”)
-
pandapower.
create_transformer_from_parameters
(net, hv_bus, lv_bus, sn_kva, vn_hv_kv, vn_lv_kv, vscr_percent, vsc_percent, pfe_kw, i0_percent, shift_degree=0, tp_side=None, tp_mid=nan, tp_max=nan, tp_min=nan, tp_st_percent=nan, tp_st_degree=nan, tp_pos=nan, tp_phase_shifter=False, in_service=True, name=None, index=None, max_loading_percent=nan, parallel=1, df=1., **kwargs)¶ Creates a two-winding transformer in table net[“trafo”]. The trafo parameters are defined through the standard type library.
- INPUT:
net - The net within this transformer should be created
hv_bus (int) - The bus on the high-voltage side on which the transformer will be connected to
lv_bus (int) - The bus on the low-voltage side on which the transformer will be connected to
sn_kva (float) - rated apparent power
vn_hv_kv (float) - rated voltage on high voltage side
vn_lv_kv (float) - rated voltage on low voltage side
vscr_percent (float) - real part of relative short-circuit voltage
vsc_percent (float) - relative short-circuit voltage
pfe_kw (float) - iron losses in kW
i0_percent (float) - open loop losses in percent of rated current
- OPTIONAL:
in_service (boolean) - True for in_service or False for out of service
parallel (integer) - number of parallel transformers
name (string) - A custom name for this transformer
shift_degree (float) - Angle shift over the transformer*
tp_side (string) - position of tap changer (“hv”, “lv”)
tp_pos (int, nan) - current tap position of the transformer. Defaults to the medium position (tp_mid)
tp_mid (int, nan) - tap position where the transformer ratio is equal to the ration of the rated voltages
tp_max (int, nan) - maximal allowed tap position
tp_min (int, nan): minimal allowed tap position
tp_st_percent (float) - tap step size for voltage magnitude in percent
tp_st_degree (float) - tap step size for voltage angle in degree*
tp_phase_shifter (bool) - whether the transformer is an ideal phase shifter*
index (int, None) - Force a specified ID if it is available. If None, the index one higher than the highest already existing index is selected.
max_loading_percent (float) - maximum current loading (only needed for OPF)
df (float) - derating factor: maximal current of transformer in relation to nominal current of transformer (from 0 to 1)
* only considered in loadflow if calculate_voltage_angles = True
- OUTPUT:
- index (int) - The unique ID of the created transformer
- EXAMPLE:
- create_transformer_from_parameters(net, hv_bus=0, lv_bus=1, name=”trafo1”, sn_kva=40, vn_hv_kv=110, vn_lv_kv=10, vsc_percent=10, vscr_percent=0.3, pfe_kw=30, i0_percent=0.1, shift_degree=30)
Input Parameters¶
net.trafo
Parameter | Datatype | Value Range | Explanation |
name | string | name of the transformer | |
std_type | string | transformer standard type name | |
hv_bus* | integer | high voltage bus index of the transformer | |
lv_bus* | integer | low voltage bus index of the transformer | |
sn_kva* | float | \(>\) 0 | rated apparent power of the transformer [kVA] |
vn_hv_kv* | float | \(>\) 0 | rated voltage at high voltage bus [kV] |
vn_lv_kv* | float | \(>\) 0 | rated voltage at low voltage bus [kV] |
vsc_percent* | float | \(>\) 0 | short circuit voltage [%] |
vscr_percent* | float | \(\geq\) 0 | real component of short circuit voltage [%] |
pfe_kw* | float | \(\geq\) 0 | iron losses [kW] |
i0_percent* | float | \(\geq\) 0 | open loop losses in [%] |
shift_degree* | float | transformer phase shift angle | |
tp_side | string | “hv”, “lv” | defines if tap changer is at the high- or low voltage side |
tp_mid | integer | rated tap position | |
tp_min | integer | minimum tap position | |
tp_max | integer | maximum tap position | |
tp_st_percent | float | \(>\) 0 | tap step size for voltage magnitude [%] |
tp_st_degree | float | \(\geq\) 0 | tap step size for voltage angle |
tp_pos | integer | current position of tap changer | |
tp_phase_shifter | bool | defines whether the transformer is an ideal phase shifter | |
parallel | int | \(>\) 0 | number of parallel transformers |
max_loading_percent** | float | \(>\) 0 | Maximum loading of the transformer with respect to sn_kva and its corresponding current at 1.0 p.u. |
df | float | 1 \(\geq\) df :math:`>`0 | derating factor: maximal current of transformer in relation to nominal current of transformer (from 0 to 1) |
in_service* | boolean | True / False | specifies if the transformer is in service. |
*necessary for executing a power flow calculation
**optimal power flow parameter
Note
The transformer loading constraint for the optimal power flow corresponds to the option trafo_loading=”current”:
Electric Model¶
The equivalent circuit used for the transformer can be set in the power flow with the parameter “trafo_model”.
trafo_model=’t’:
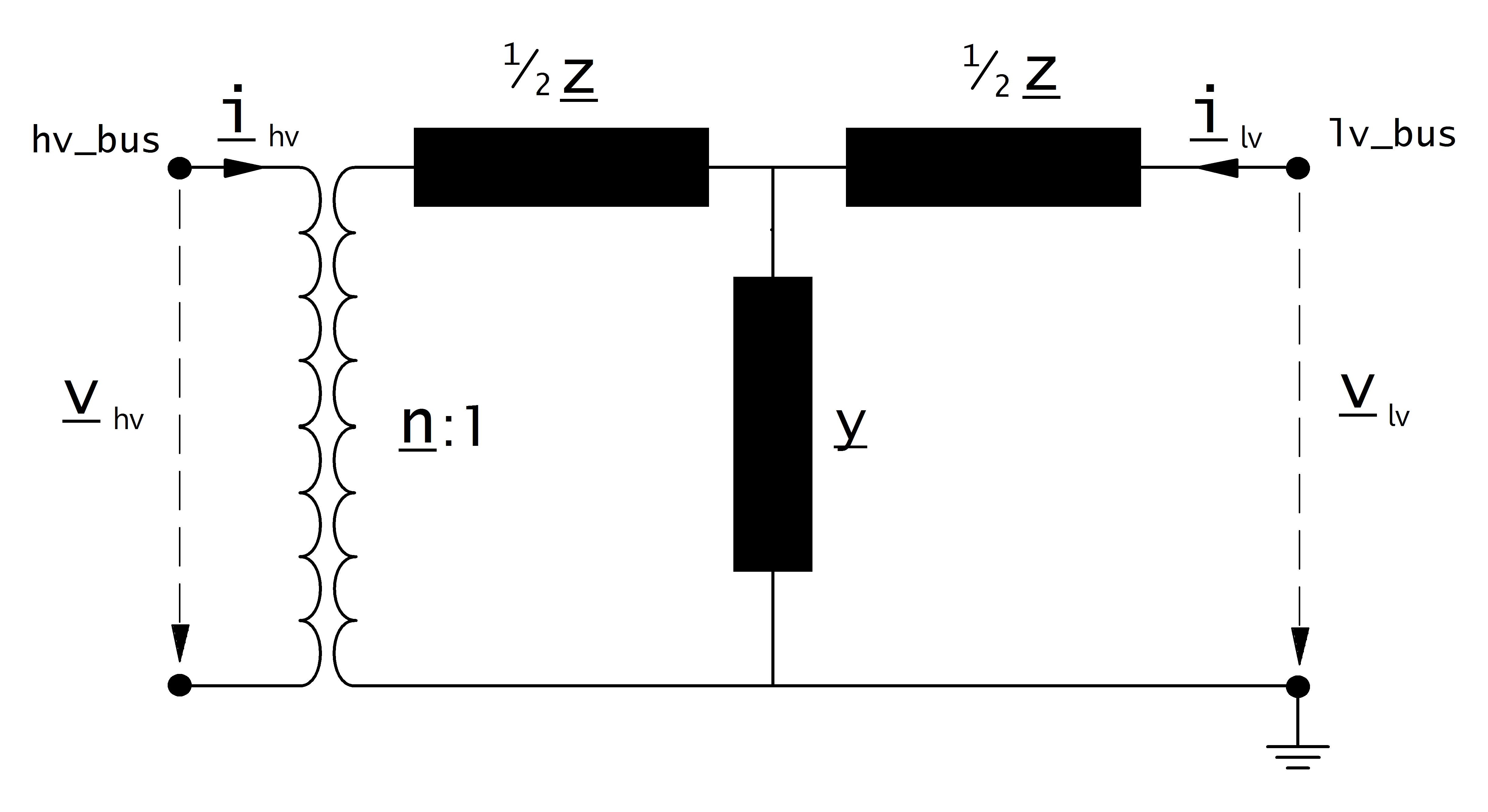
trafo_model=’pi’:
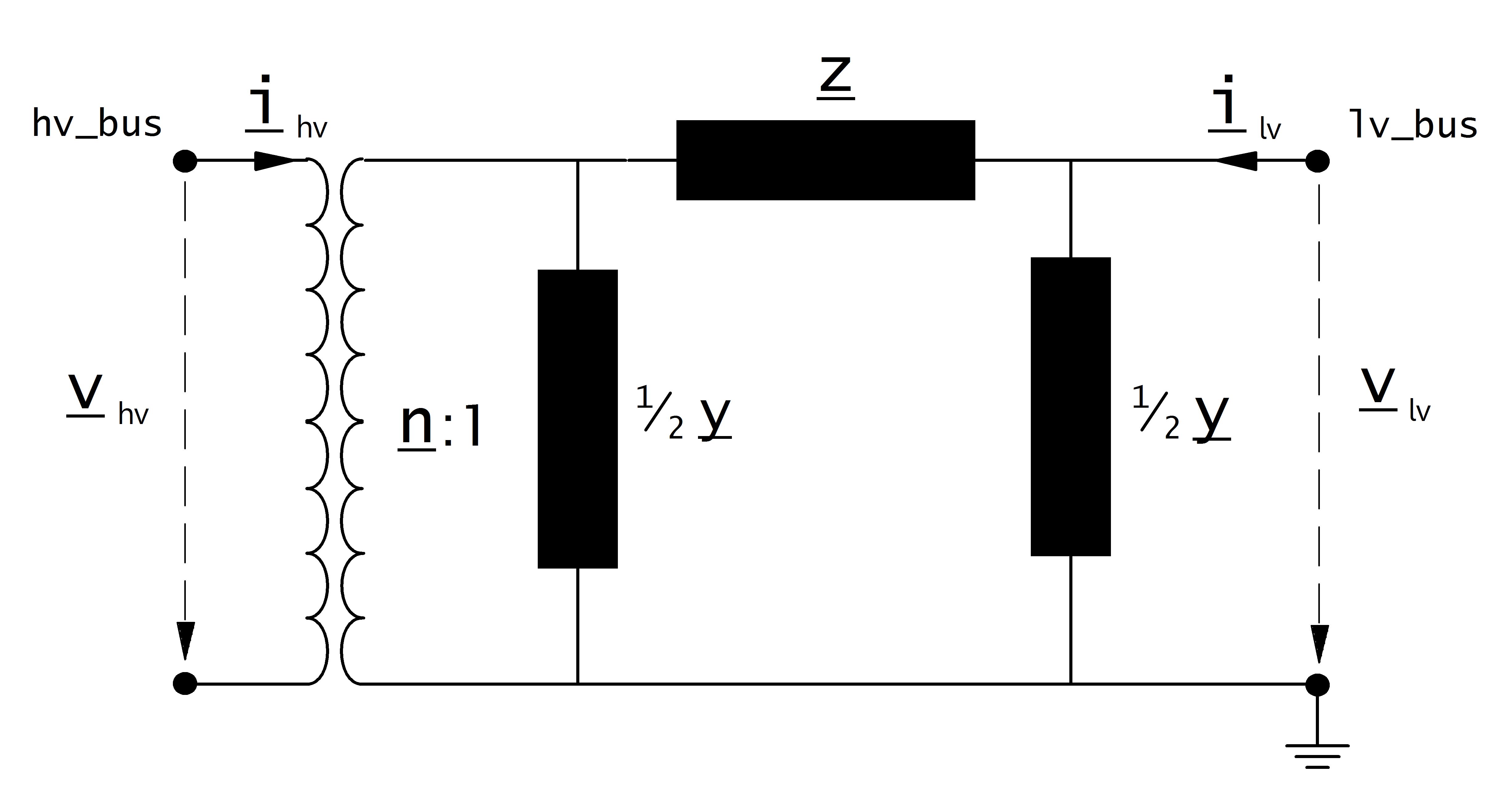
Transformer Ratio¶
The magnitude of the transformer ratio is given as:
The reference voltages of the high- and low voltage buses are taken from the net.bus table. The reference voltage of the transformer is taken directly from the transformer table:
If the power flow is run with voltage_angles=True, the complex ratio is given as:
Otherwise, the ratio does not include a phase shift:
Impedance Values¶
The short-circuit impedance is calculated as:
The magnetising admittance is calculated as:
The values calculated in that way are relative to the rated values of the transformer. To transform them into the per unit system, they have to be converted to the rated values of the network:
Where the reference voltage \(V_{N}\) is the nominal voltage at the low voltage side of the transformer and the rated apparent power \(S_{N}\) is defined system wide in the net object (see Unit Systems and Conventions).
Tap Changer¶
Longitudinal regulator
A longitudinal regulator can be modeled by setting tp_phase_shifter to False and defining the tap changer voltage step with tp_st_percent.
The reference voltage is then multiplied with the tap factor:
On which side the reference voltage is adapted depends on the \(tp\_side\) variable:
tp_side=”hv” | tp_side=”lv” | |
\(V_{n, HV, transformer}\) | \(vnh\_kv \cdot n_{tap}\) | \(vnh\_kv\) |
\(V_{n, LV, transformer}\) | \(vnl\_kv\) | \(vnl\_kv \cdot n_{tap}\) |
Note
The variables tp_min and tp_max are not considered in the power flow. The user is responsible to ensure that tp_min < tp_pos < tp_max!
Cross regulator
In addition to tp_st_percent a value for tp_st_degree can be defined to model an angle shift for each tap, resulting in a cross regulator that affects the magnitude as well as the angle of the transformer ratio.
Ideal phase shifter
If tp_phase_shifter is set to True, the tap changer is modeled as an ideal phase shifter, meaning that a constant angle shift is added with each tap step:
The angle shift can be directly defined in tp_st_degree, in which case:
or it can be given as a constant voltage step in tp_st_percent, in which case the angle is calculated as:
If both values are given for an ideal phase shift transformer, the power flow will raise an error.
Result Parameters¶
net.res_trafo
Parameter | Datatype | Explanation |
p_hv_kw | float | active power flow at the high voltage transformer bus [kW] |
q_hv_kvar | float | reactive power flow at the high voltage transformer bus [kVar] |
p_lv_kw | float | active power flow at the low voltage transformer bus [kW] |
q_lv_kvar | float | reactive power flow at the low voltage transformer bus [kVar] |
pl_kw | float | active power losses of the transformer [kW] |
ql_kvar | float | reactive power consumption of the transformer [kvar] |
i_hv_ka | float | current at the high voltage side of the transformer [kA] |
i_lv_ka | float | current at the low voltage side of the transformer [kA] |
loading_percent | float | load utilization relative to rated power [%] |
The definition of the transformer loading depends on the trafo_loading parameter of the power flow.
For trafo_loading=”current”, the loading is calculated as:
For trafo_loading=”power”, the loading is defined as: